Navigation Panel
Overview
Navigation Panel displays a list of links and link groups divided in categories for registered Navigation Panel items that allow user to navigate to different entry stations within the solution.
Based on which view user is located Navigation Panel is either docked to the left side of the view (in case of home view) or hidden under hamburger menu button.
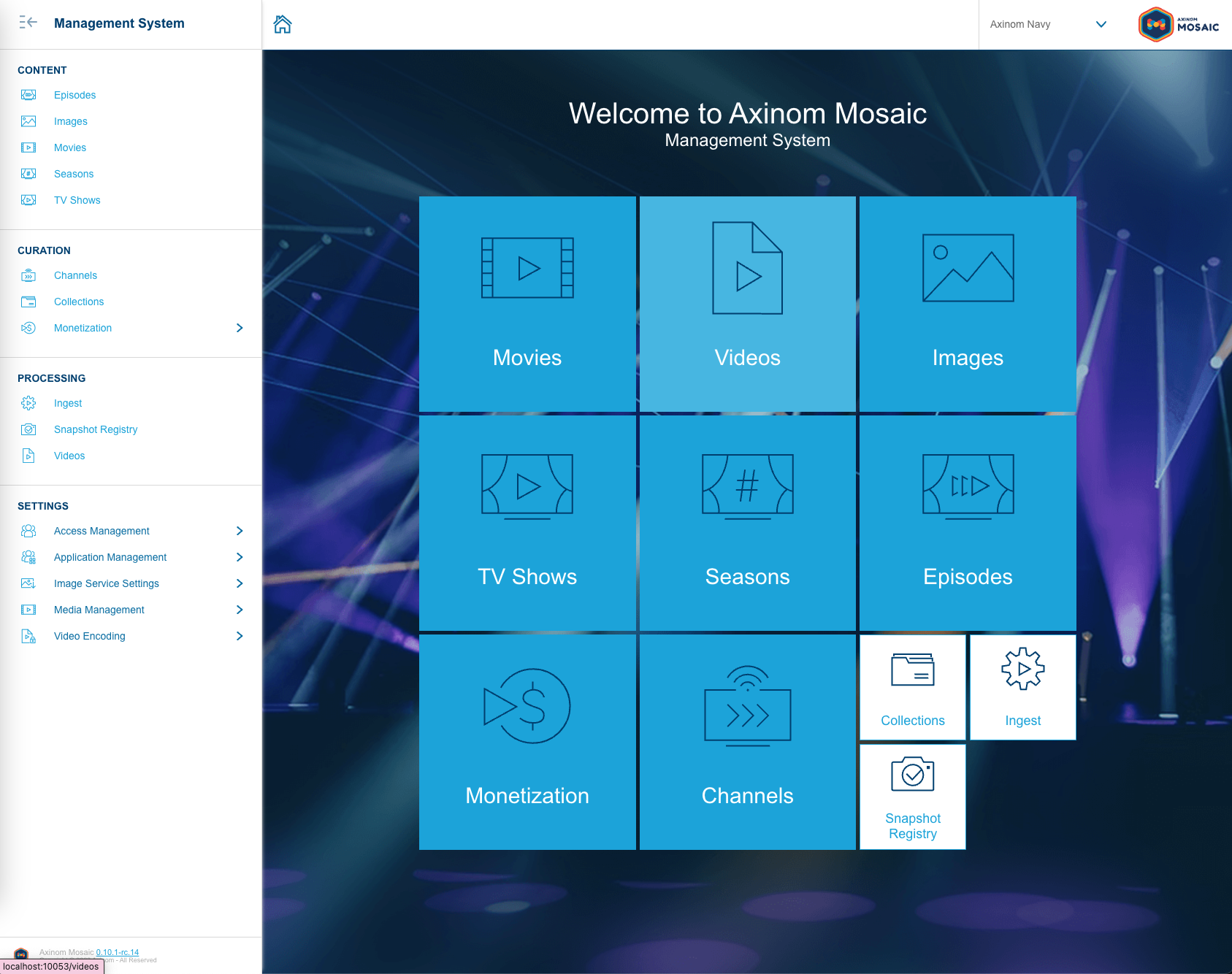
By default all links that are registered with the Pilet API registerTile
method are added to the Navigation Panel, if not specified otherwise.
Pilet API contains methods to register Navigation Panel items and customize existing ones with transformers, e.g. rename, reorder, change grouping.
Managed Solution adds Navigation Panel items in two categories Workflows and Settings. Mosaic Media Template registers a transformer that distribute Workflows category items to other customizable solution categories.
Register Navigation Panel Item
For new Navigation Panel item registration Pilet API contains a method registerNavigationItem
.
Here’s an example of new navigation item registration:
import { IconName, Icons } from '@axinom/mosaic-ui';
function register(app: PiletApi) {
// ...
app.registerNavigationItem({
categoryName: 'My Category',
icon: <Icons icon={IconName.Info} />,
label: 'New Navigation Group',
name: 'new-navigation-group',
});
app.registerNavigationItem({
categoryName: 'My Category',
icon: <Icons icon={IconName.Info} />,
label: 'New Navigation Item',
name: 'new-navigation-item',
parentName: 'new-navigation-group',
path: '/new-settings-item',
});
}
The first registerNavigationItem
call in example adds parent group in new category with name My Category .
The next call adds a child item to this group, notice that parentName
in registration call needs to match parent groups name
for it to be placed in this group.
In case home view tile is also registered with registerTile
call for the new navigation item, to disable automatic Navigation Panel item registration, second argument with value false
needs to be passed in registerTile
call like shown in the following example:
app.registerTile({
kind: 'home',
label: 'New Navigation Item',
name: 'new-setting',
path: '/new-settings-item',
type: 'large',
}, false);
Navigation Panel Transformers
Transformers can be set to modify existing navigation panel items, their order and grouping. Piral API provides two setter methods to set transformer functions setNavigationItemsTransformer
and setNavigationTreeTransformer
.
setNavigationItemsTransformer
The first one setNavigationItemsTransformer
accepts as argument function that will receive flat array of registered navigation item objects and it must return transformed navigation item array that will be used to create Navigation Panel categories and navigation tree.
This transformer offers option to easily modify individual navigation items, change child item grouping, add completely new groups or change item categories.
Example use of items transformer:
function register(app: PiletApi) {
// ...
function transformNavigationItems(items: NavigationItem[]): NavigationItem[] {
items.forEach((item) => {
// move managed solution 'WORKFLOWS' category to our newly created category
if (item.categoryName.toLowerCase() === 'workflows') {
item.categoryName = 'My Category';
}
});
// sort navigation items in reverse order by label
items
.sort((a, b) => String(a.label).localeCompare(String(b.label)))
.reverse();
return items;
}
app.setNavigationItemsTransformer(transformNavigationItems);
}
In the example transformer renames workflows
category to content
and then sorts items in reverse order by label.
Note
|
In Mosaic Media Template there is already a transformer added that moves the items from workflows category to other categories. When adding your custom sorter you should remove this sorter by removing it’s registration call setNavigationItemsTransformer in file located at services/media/workflows/stc/index.tsx . When multiple transformers are registered only last one registered will be applied.
|
setNavigationTreeTransformer
The second transformer setNavigationTreeTransformer
sets a transformer function that will receive navigation tree, that was created from registered navigation items.
This transformer can be used to add custom sorters for navigation item groups and categories, sort and reorder categories in Navigation Panel.
The default transformations that are applied is alphabetical sorting of categories by categoryName
and moving the Settings category as a last item.
When setting custom transformer these transformations will be overwritten.
Here’s an example of navigation tree transformer:
function register(app: PiletApi) {
// ...
function transformNavigationItemTree(
navTreeCategories: NavigationPanelCategory[],
): NavigationPanelCategory[] {
// sort categories alphabetically
navTreeCategories.sort((a, b) =>
String(a.categoryName).localeCompare(String(b.categoryName)),
);
// move 'my category' as first category in the navigation
const catIndex = navTreeCategories.findIndex(
(item) => item.categoryName.toLowerCase() === 'my category',
);
navTreeCategories.unshift(navTreeCategories.splice(catIndex, 1)[0]);
return navTreeCategories;
}
app.setNavigationTreeTransformer(transformNavigationItemTree);
}
Navigation tree transformation function in given example sorts categories alphabetically by name and moves category with name My Category to the beginning or the navigation tree in Navigation Panel.
After registering new navigation items and applying described transformations you should be able to see the updated Navigation Panel arrangement similar like shown in image below.
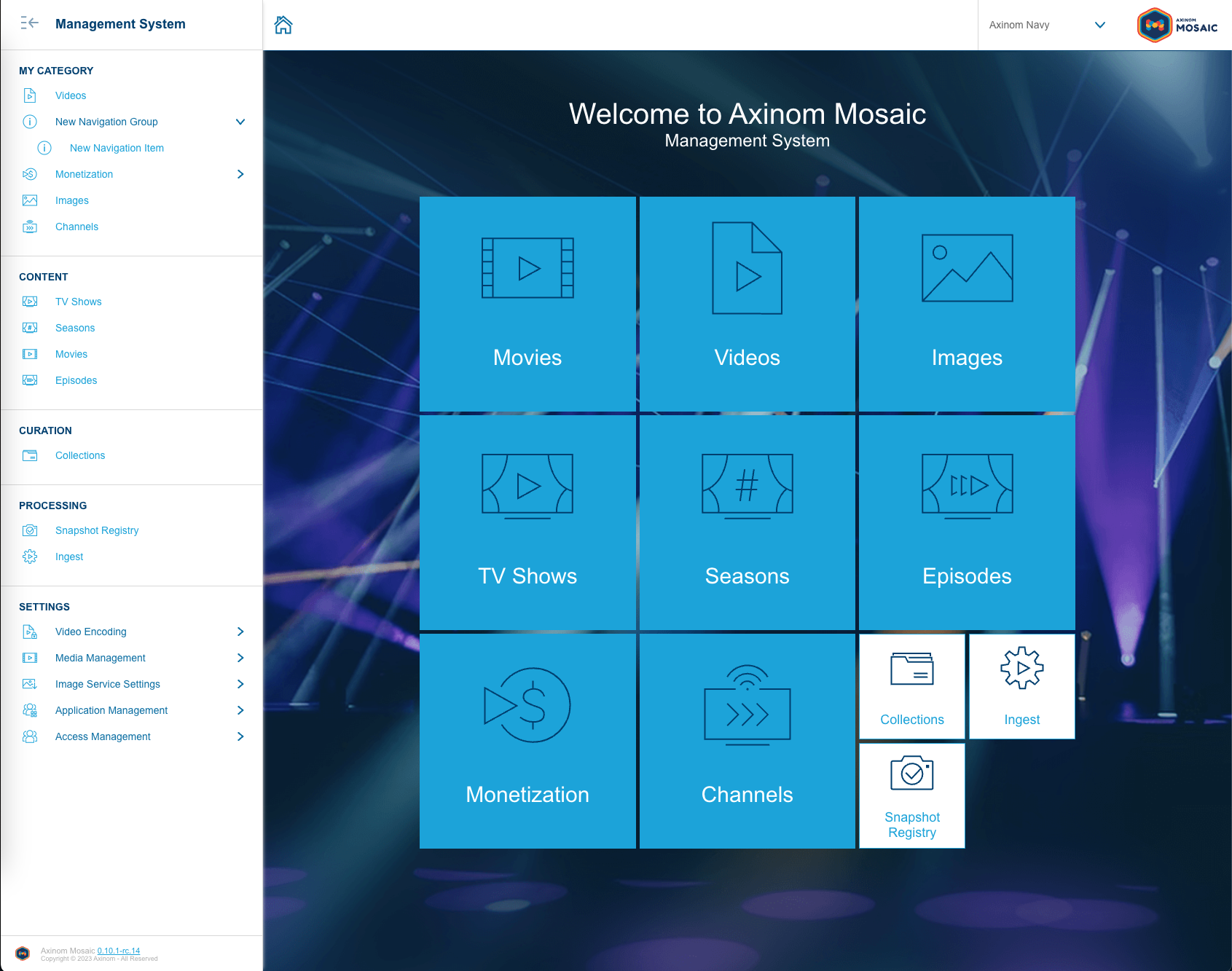